@forbzie22_0253 you can use the same ProGet Free on multiple instances
Welcome to the Inedo Forums! Check out the Forums Guide for help getting started.
If you are experiencing any issues with the forum software, please visit the Contact Form on our website and let us know!
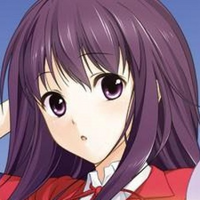
Posts made by atripp
-
RE: Apply License via API
-
RE: |OTTER] Status filter not working for "any error"
Thanks for the bug report @philippe-camelio_3885 !
I logged as OT-508 , and we'll target it an upcoming maintenance release :)
-
RE: ProGet Enteprise License Update
Hi @scott-wright_8356 ,
You can ignore that message; not sure how/why it occurred, but the consequence is that the message that the license key was updated wasn't immediately dispatched to the other nodes due to some timint issue. So that means it could take a few minutes for the other nodes to reflect the new license key.
We should probably just ignore that error. let us know if you keep seeing "No primary service node has been registered." and we can investigate further.
Best,
Alana -
RE: VulnerabilityDownloader fails with error
Thanks @jw, we still aren't quite sure what's behind this, but we rewrote
PgvdVulnerabilities_UpdateIndex
procedure to run much faster:This will be included in ProGet 2023.32 via PG-2615
I just published ProGet 2023.32-rc.1 as a pre-release ; would you mind to try it out on your test server, since you can reproduce it?
https://docs.inedo.com/docs/howto-install-prerelease-product-versions
-
RE: Unable to add license to package
Hi @v-makkenze_6348 ,
I wasn't sure what version you're on. I'm not able to reproduce this in ProGet 2023.32, so I wonder if it's a possibly a bug in an earlier version of ProGet 2023? We recently made some fixes.
How did you try to add the license as Package name?
Did you manually edit the license, or use the dialog to add the entry?
Thanks,
Alana -
RE: Timeout in build when running pgscan
Hi @v-makkenze_6348,
Just want to confirm here -- it sounds like it's working, except the issue now that you're only seeing 634 packages but not 363?
One thing I suspect is that one of the packageurls in the SBOM is invalid (incorrect format). Are you able to find which item isn't being imported?
It might be missing a version or something else?
Thanks,
Alana -
RE: VulnerabilityDownloader fails with error
@jw That is so weird.
Is it always failing with the follow?
Unable to read data from the transport connection: An existing connection was forcibly closed by the remote host..
Looking over your Prod dates... did you happen to upgrade after 2024.03.14? Or maybe that's when you enabled the preview feature?
Could you try running
sp_updatestats
and cleaning fragmentation on those tables usig SQL Management Studio? Clearly there's something we're missing here...Thanks,
Alana -
RE: Lots of Powershell and Conhost processes
Hi @Justinvolved ,
This is not uncommon with PowerShell; in general the processes will be closed, but there are enough scenarios with long-running/hanging PowerShell scripts where those processes will report termination but the process will not be terminated. So they can stick around
I'd recommend using the Inedo Agent instead, even if it's on the same sever. That gives better process isolation compared to the local agent, and you shouldn't see this behavior for too long. The local agent runs in-process.
Cheers,
Alana -
RE: VulnerabilityDownloader fails with error
Hi @jw ,
This job should take like a minute tops. It's inserting/updating about 50k vulnerability records (
PgvdVulnerabilities
) and maybe 200k related package records (PgvdPackageNames
). The later packages tend to have more related packages, but not that many. In either case that's not a lot for SQL Server.Any help in troubleshooting would be appreciated, since we're kind of at a loss. Behind the scenes, the
PgvdVulnerabilities_UpdateIndex
procedure is being called with batches of 1000 vulnerability records, and it's exclusively locking both tables.We noticed that micro-instances of SQL Server (e.g. 1 core / 512MB ram) would fail without batching, but otherwise it completes in about 5 minutes. I assume you're not running that?
One user reported an unpatched SQL 2016 had a severe performance issue with an
OUTPUT
clause we were using, so we stopped using that. Now it works in that version.Maybe it's another SQL Server bug? Or perhaps something we're otherwise missing?
Maybe a forced
sp_updatestats
would do the trick? Or cleaning up fragmentation on those tables (again, shouldn't be a problem....)Any insight would be helpful. We don't know how to rewrite this any more effectively, and batches of 1000 seem about as small as we want to make them.
Thanks,
Alana -
RE: Proget Python connector to PyTorch Cuda Index
Hi @m-karing_2439 ,
Unfortunately, this repository doesn't following PyPI repository conventions.
For example:
- No way to see latest packages (should be https://download.pytorch.org/whl/cu118/rss/updates.xml)
- Does not return anything for
/simple
listing (e.g. https://download.pytorch.org/whl/cu118/simple) - Does not support JSON-based API to retrieve package metatadata
I guess it "happens to work" in pip , or perhaps they install a plugin that allows it to work... but ProGet is designed around PyPi repository specs. which is why a non-confirming download site won't work very well.
I'll add a note to investigate this further, but for the time being you should just download the wheels and upload them to a feed in ProGet.Cheers,
Alana -
RE: Maven Andriod Endpoint URL
I looked into this further; for Maven feeds, the Connector Health Check simply queries the downloaded index. If there is no downloaded index, then it reports back as healthy. If there's an error with the index, it reports an error. However, most maven repositories don't have a downloadable index.
Anyway the health check is not very useful, and we plan to revisit the functionality in the future, since there's really no way to determine if ProGet can download an artifact unless you know the exact name.
Thanks,
Alana -
RE: SQL Server execution timeouts after updating to 2023.31 (Build 5)
@appplat_4310 thanks for the update!
We also applied the patch to the next maintenance release, so you shouldn't have any issues going forward then!
-
RE: Maven Andriod Endpoint URL
It's a bit hard to figure out how to help or troubleshoot with the information here, but a few key points:
- A "healthy" Maven connector doesn't really mean much; just that the URL can be accessed by the ProGet server.
- A Maven repository (like the one you are linking to) is just a "web file system" with url/folder based conventions
- A Maven "package" consists of a .pom file and zero or more other files (.jar); think of it liked an unzipped package that you can add/delete files from
- This is why you can't import only .jar files - you need the .pom file (which serves as the package manifest)
- Sometime marvin "packages" contain malformed .pom files that happen to work in some versions of the client, and ProGet will sometimes error on reading those; maybe that's what happening here
To help on this, we'll need to know specifically where the issue is and have instructions on how to reproduce it on a new feed/instance of ProGet.
First, I would start by trying to download the .pom file and .jar files from Google (like that gradle one you found), then upload them to ProGet from the Web UI. If that doesn't work, then we know the POM file is probably bad, and we can investigate it.
Next, I would try to pull the pom/jar from a connector. If that doesn't work (but uploading it does), then maybe the "API" for that repository is incorrect. And we can investigate it.
Best,
Alana -
RE: Maven Andriod Endpoint URL
Hi @scott-wright_8356 ,
I'm not sure, but based on this URL:
https://maven.google.com/web/index.html#android.arch.core:common:1.1.1I can see that the POM for
android.arch.core.common 1.1.1
is located here:
https://dl.google.com/android/maven2/android/arch/core/common/1.1.1/common-1.1.1.pomSo the base URL would be:
https://dl.google.com/android/maven2That should work, since maven works by convention. You will not see a listing of artifacts unfortunately, since Maven does not provide an index. You just need to know the articats by name, then append maven-metadata.xml.
As expected, the metadata file for that artifact is here:
https://dl.google.com/android/maven2/android/arch/core/common/maven-metadata.xmlCheers,
Alana -
RE: ProGet dropPath for package imports
Hi @forbzie22_0253 , that's only possible in the one-off GUI option. It's not possible if you're using a drop path
-
RE: Database Error
Hello, just letting you know we're aware of the issue and plan to investigate/fix soon! And we hope then to get your feedback on the preview feature :)
-
RE: Maven feed for cache
Hi @scott-wright_8356 ,
Thanks; it sounds like the underlying issue is indeed Google Android connector you added. I don't have an information or idea on why that might be the case... some third-party repos (Maven, PyPi, NuGet, etc.) are just really slow and buggy.
ProGet is basically acting like a proxy server here, so "garbage in, garbage out" applies - if the connector is slow and error-prone, then you will experience slowness and errors.
Sometimes third-party repos will create packages that don't follow the specifications but just happen to work in certain versions of clients. That could be what's happening here. In cases like that, we can really only address those on a case-by-case basis, and we'd need to know exactly how to reproduce the error and investigate it later.
Best,
Alana -
RE: ProGet dropPath for package imports
The use case you're describing (i.e. using drop paths as an intermediate to publish packages to ProGet) is not uncommon. It's fine, and can be simpler in many cases.
But since you asked... the downsides are that require file share access, new developers may be confused by the process (since the typical workflow is publishing directly), and that there is a lack of immediate feedback mechanism (just because file copy is success doesn't mean package is accepted). I guess those are all obvious.
As to your other questions...
Can ProGet handle situations where multiple computers are trying to install a module from the feed while at the same time that module is being updated by the dropPath feature?
Package versions are immutable and not meant to be overwritten. Instead you are supposed to publish new versions. If your workflow involves continuously overwriting the same version while continuously consuming it, you will get errors. Regardless of using drop paths or publishing.
Could these be potential file locking situations where a package is copied to the drop path and ProGet is trying to access it in the dropPath?
If the file in a drop path is locked for reading (since it's currently being written), ProGet will just try again later.
-
RE: Maven feed for cache
Hi @scott-wright_8356 ,
Can you try downgrading to 2023.30? I see some Maven changes, and any time there's a possibility of a regression. That will at least tell us where the issue might be.
Thanks,
Alana -
RE: ProGet IIS Application Pool Managed Pipeline Mode
Hi @scott-wright_8356 ,
You can change if you'd like, I'm not sure if it makes any difference with .NET6+ applications.
Cheers,
Alana -
RE: SQL Server execution timeouts after updating to 2023.31 (Build 5)
Hi @appplat_4310 ,
In 2023.31, we made made introduced various database performance improvements (deadlock, timeout reductions) via PG-2606, so it's possibly related to that. The query you identified most definitely helped, and it there's only one place it's called.
WE would be super-grateful if you could try running this?
ALTER PROCEDURE [NuGet_GetPackage] ( @Feed_Id INT, @Package_Id VARCHAR(255), @Version_Text VARCHAR(255) = NULL ) AS BEGIN SET NOCOUNT ON SELECT * FROM [NuGetFeedPackageVersions_Extended] WHERE [Feed_Id] = @Feed_Id AND [PackageGroup_Name] IS NULL AND [PackageType_Name] = 'nuget' AND [Package_Name_Lower] = LOWER(@Package_Id) AND (@Version_Text IS NULL OR [Package_Version] = @Version_Text) END
We believe that, for some reason, your query analyzer is following a different plan, but the new conditionals should force it to use the right index.
Let us know, and we'll get it fixed right away.
Thanks,
Alana -
RE: ProGet 2023 - IIS App pool stopping
Hi @rick-kramer_9238 , you could certainly try to enable those and see if they help or make a change.
-
RE: SQL Error after upgrade version
@scott-wright_8356 the Inedo Hub will upgrade the database code (stored procs, views) when doing an installation. So from the time you upgrade the first server to the last server of a cluster, the "database code" and "server code" will be "out of sync", yielding errors like this.
For most users it's okay, and they will typically (manually) upgrade all nodes over the course of a few minutes during a mtaintenance period etc.
-
RE: ProGet SCA - License files
Hi @jw , thanks for pointing this out! I added a note to review/investigate this in our run-up to finalizing ProGet 2024.
-
RE: [OTTER]Gitlab Secure Ressource gone
Without looking closer or testing it's hard to say, but that error sounds like the property isn't being set? You can see rom the the source code of Git::Checkout-Code, the
BranchOrCommit
argument (calledObjectish
in the code) will useDefaultValue
of$commit
unless a property is specified .I guess just as a test, does
set $commit = master
make any difference?Thanks,
Alana -
RE: ProGet 2023 - IIS App pool stopping
Hi @rick-kramer_9238 ,
It's possible that the IIS App-pool is automatically shutting down, which can lead to a "warm up" time that's required on a first request. However, this is typically measured in seconds in slow cases, not minutes. So it shouldn't take that long to "warm up".
Unfortunately this isn't easy to troubleshoot, and it seems to be related to some kind of strange IIS configuration. We've heard of users solving it lots of different ways, from switching servers to uninstalling IIS, to switching to the Integrated Web Server. But no idea what actually works.
The easiest thing to do, you may want to consider switching to the Integrated Web Server. Microsoft recommends that over IIS these days as well. The quickest way to do this is to uninstall ProGet (this does not delete database or packages), then reinstall to use the integrated web server. Make sure to point to same database at install time. You can configure HTTPS later.
Best,
Alana -
RE: SQL Server permissions issue in ProGet installation
Hi @gurdip-sira_1271 ,
That message means that the Login for
GLOBAL\GLOPROGET01$
does not exist at the SQL Server level; you can add this in SQL Server Management Stuiod, under Security > Logins.Cheers,
Alana -
RE: ProGet 2023 - IIS App pool stopping
Hello,
I am copy-pasting the same reply made to EDO-10211 -- feel free to reply to one or the other.
We'll do our best to help, but based on the information you provided, it sounds like your server might be "overloaded" and have more traffic than it can handle. I would recommend trying out ProGet 2023.31 (releasing later today) which has a few more performance tweaks, but if that doesn't solve the issue than it will involve looking at ways to expand server/clsuter capacity or reduce/throttle traffic.
The error you shared is unfortunately unrelated, and is just a generic "client disconnected" error - it will occur if a a client (web browser, etc) disconnects prior a request being received. These should not be logged, but due to a known issue in .NET6 they are. This should be fixed in .NET8 (ProGet 2024).
Best place to check is ProGet Diagnostic Center, under Admin. That will have the most relavent errors.
Best,
Alana -
RE: Create Feed via API Timeout
Hi @forbzie22_0253 ,
(Sorry this is basically a copy/paste of the same answer to @philippe-camelio_3885 's question)
We haven't run into any of these issues in our testing, but this error is a "generic database timeout", which is implying that "something" is going on with the database. That's the only information we have, "something".
To troubleshoot this, you'll need to use some of SQL Server's performance/activity monitoring tools to spot what's going on at the time. It could be anything from outdated statistics to a missing index to a bad query. Or who knows.
With ProGet, timeouts can happen during extremely high usage of feeds/server. We have also seen instances where SQL Server's auto-statistics aren't working, and rebooting the server will help -- you can try running
sp_UpdateStatistics
as well. I have never seen that personally, but two users reported this already.Best,
Alana -
RE: [OTTER] Create new server thru API hangs
We haven't run into any of these issues in our testing, but this error is a "generic database timeout", which is implying that "something" is going on with the database. That's the only information we have, "something".
To troubleshoot this, you'll need to use some of SQL Server's performance/activity monitoring tools to spot what's going on at the time. It could be anything from outdated statistics to a missing index to a bad query. Or who knows.
Best,
Alana -
RE: Reverse proxy - otter behind path
We do not support "sub-path content/URL Rewriting" in any of our products and strongly discourage even trying. It won't work and will just lead to lots of wasted time and headaches, since everything from javascript ajax requests to CSS background images to cookies assumes a well-known root path of
/
for the application.Best,
Alana -
RE: [OTTER]Gitlab Secure Ressource gone
In BuildMaster 2022, we redesigned the Git and Source Control experience, which required significant changes to the various Git extensions (Git, GitHub, GitLab, and AzureDevOps).
With these changes, instead of using service-specific operations like
GitHub::Get-Source
, you can simply use operations likeGit::Checkout-Code
. Behind the scenes, BuildMaster wires everything up using build variables like$Repository
and$Commit
.In Otter, you'll need to specify those variables or
To
andBranchOrCommit
properties, similar to this:Git::Checkout-Code ( To: $DossierVisHab, From: gitlab-vishab BranchOrCommit: master, );
The extensions are indeed the same between BuildMAster and Otter, but they do different things than before. Now, the
GitLab
extension mostly just provides integration into Issue Tracking, and information intended for the BuildMaster UI, such as a list of organizations, repositories, etc.Best,
Alana -
RE: [OTTER]Gitlab Secure Ressource gone
I didn't realize it was possible to create those in Otter
I thought only Generic Git repositories work. There's no GitLab/Github/etc.-specific functionality available in Otter, those are primarily intended for BuildMaster's integrations.
I would just use Generic Git repository, since the Git-base doperations will all be the same.
Cheers,
Alana -
RE: Cloud Package Storage and Local Reads
Hi @mahdy-merry_6333 ,
It's possible, and you can do this by creating a second feed that effectively proxies the first feed and uses disk storage. You could use a Connector on the two feeds or REplication.
Cheers,
Alana -
RE: Trial License Request Error
Hi @matt-wood_5559 ,
Looks like your account was added to My Inedo incorrectly due to a human error (someone manually added it on our end since you had been talking to sales)... but it looks like its already been corrected, and you have a working key to use now. You shouldn't have any issues going forward.
Cheers,
Alana -
RE: ProGet Packages error getting records
@appplat_4310 yes please :)
Once I get confirmation it works, i'll commit the changes so it goes in the next maintenance release
-
RE: Timeout in build when running pgscan
It's really hard to say, but that's probably it. We'd really need to analyze it further to tell. How many packages are in the release?
FYI - this is all getting a "total rewrite" in ProGet 2024, and you can preview some of the features in the latest release of PRoGet
-
RE: Cannot recreate deleted Asset SubFolder
Hi @norm-ross_1437 ,
I'm not sure what version of ProGet you're using, but this sounds familiar and I'm almost certain we fixed this specific case a while ago. So I would try to upgrade.
I don't realy recommend playing around with data in the tables....
Best,
Alana -
RE: Timeout in build when running pgscan
Hi @v-makkenze_6348 ,
I'm not sure what version you upgraded from, but there hasn't really been many changes that would have caused this I think.
This is a general database timeout error, which could be happening under heavy load (so trying again should work), or it could be a result of needing to update statistics, etc.
Thanks,
Alana -
RE: SQL Error after upgrade version
@lucas-almeida_8120 in that case, I would use a import path - https://docs.inedo.com/docs/proget-bulk-import-with-droppath
-
RE: ProGet Packages error getting records
Hi @scott-wright_8356 ,
Thanks for sharing that; I think I see where the issue is.
This is recent regression... can you run the
1.FeedPackageVersions_ExtendedWithMaven.sql
script that's attached to PG-2604? I think that will fix the issue.Thanks,
Alana -
RE: ProGet Packages error getting records
Hi @scott-wright_8356 ,
That's strange; I'm not sure how that's possible. There's clearly some bad/unexpected data in the maven tables, and I'm not sure how that happened. Maybe an error/bad API call? I don't know.
Can you help us identify this by running
EXEC Packages_GetPackages
orSELECT * FROM
FeedPackageVersions_ExtendedWithMaven, and seeing if you can spot any rows with with a null
Package_Name`?Specifically, I'm looking for something that would cause this below function to crash. The only thing I can think of is a null
Package_Name
.public static string BuildUrl(FeedId feedId, string group, string name, string version) { var builder = new StringBuilder(); builder.Append("/feeds/"); builder.Append(Uri.EscapeDataString(feedId.Name)); builder.Append('/'); if (!string.IsNullOrWhiteSpace(group)) { builder.Append(Uri.EscapeUriString(group)); builder.Append('/'); } builder.Append(Uri.EscapeDataString(name)); if (!string.IsNullOrEmpty(version)) { builder.Append('/'); builder.Append(Uri.EscapeDataString(version)); } return builder.ToString(); }
-
RE: Programmatic way to manage API keys
Hi @forbzie22_0253 ,
We are aiming to release ProGet 2024 in early April; here are the upgrade notes in progress, which will give some idea of what's coming: https://docs.inedo.com/docs/proget-upgrade-2024
Best,
Alana -
RE: SQL Error after upgrade version
Hi @lucas-almeida_8120 ,
This error is indicating that your database not being upgraded along with the rest of your installation. Or is otherwise corrupt. I can't imagine how that could happen, so unfortunately you'll have to do some digging on this.
Here is where the connection string for the ProGet database is stored:
https://docs.inedo.com/docs/installation-configuration-filesIf you review the installation logs in the Inedo Hub, you will see what database/connectionstring is being used.
Good luck, and let us know what you find!
Alana
-
RE: ProGet Cluster Configuration Info
This is usually due to a networking error; it may also be resolved by restarting. It's also just something you can ignore. The service messanger isn't very important in ProGet.
Best,
Alana -
RE: ProGet HA Availability Setup and Update
When installing ProGet with the Inedo Hub, you can specify the SQL Server and Database Name. If it's an existing ProGet database, then it will be upgraded/downgraded as needed. I'm don't know what the errors were, but typically the installing users has a lack of db_owner permissions.
To upgrade ProGet in an HA set-up, you can just use the Inedo Hub to upgrade each node. Usually it takes 1-2 minutes per node, so you can do it manually pretty easily. There shouldn't be any errors.
Best,
Alana -
RE: ProGet npm feed Errors in High Availability structure
Hi @scott-wright_8356 ,
This error means that "something" that is taking an exclusive lock handle on files within your SMBShare. Unfortunately, the operating system does not provide information about what that "something" is, but it's typically an anti-virus, search-indexing, or back-up tool.
ProGet uses shared read handles, but obviously needs a lock handle when writing. There are a few race conditions where this could occur, but they are extremely rare and require multiple requests on different servers in a cluster that attempt to add the same package at the same time.
However those are very rare, so I suspect "something else" is locking those files. Usually it's an anti-virus tool - since it "looks like" a zip file, it's being opened? You'd need to use a tool to monitor openarting system handles, like sysinternals procmon.
Best,
Alana -
RE: Programmatic way to manage API keys
Hi @forbzie22_0253 ,
We do not currently have an API Key API, but this is something we are planning for ProGet 2024.
Today, you'd need to use the Native API. Since you're automating an installation, it's easeist to just use SQL to call those API/Stored Procs, since you'll also want to modify other properties like license key, etc.
Best,
Alana